
Containers are essential SWING GUI components. A container is a space in which a component can be placed. A Container in AWT is a component in and of itself, with the ability to add another component to it.
Containers at the highest level:
- It inherits AWT’s Component and Container.
- It can’t be contained by anything else.
- Heavyweight.
- JFrame, JDialog, and JApplet are examples.
Lightweight containers:
- It derives from the JComponent class.
- It is a multipurpose container.
- Furthermore, it can be used to group related components.
- JPanel is an example.
Java JButton:
The JButton class is used to generate a labeled button with platform independence. When the button is pressed, the application performs some action. It derives from the AbstractButton class.
Example of Java JButton Example with ActionListener:
package gui1;
import java.awt.EventQueue;
import javax.swing.JFrame;
import javax.swing.JOptionPane;
import net.miginfocom.swing.MigLayout;
import javax.swing.JButton;
import java.awt.event.ActionListener;
import java.awt.event.ActionEvent;
public class testswing {
private JFrame frame;
/**
* Launch the application.
*/
public static void main(String[] args) {
EventQueue.invokeLater(new Runnable() {
public void run() {
try {
testswing window = new testswing();
window.frame.setVisible(true);
} catch (Exception e) {
e.printStackTrace();
}
}
});
}
/**
* Create the application.
*/
public testswing() {
initialize();
}
/**
* Initialize the contents of the frame.
*/
private void initialize() {
frame = new JFrame("Button Example");
frame.setBounds(100, 100, 450, 300);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.getContentPane().setLayout(new MigLayout("", "[][][][][][][][]", "[][][][]"));
JButton btnClickMe = new JButton("CLICK ME");
btnClickMe.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
JOptionPane.showMessageDialog(null, "button clicked");
}
});
frame.getContentPane().add(btnClickMe, "cell 6 3");
}
}
Output:
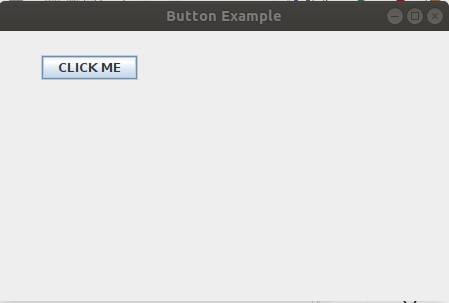
When the button is clicked then a message dialogue box pops up.
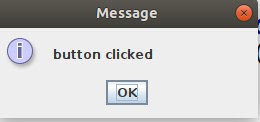
Java JTextField:
A JTextField class object is a text component that enables for the editing of a single line of text. It derives from the JTextComponent class.
Constructors:
JTextField()
JTextField(String text)
JTextField(String text, int columns)
JTextField(int columns)
Java JCheckbox:
To construct a checkbox, utilize the JCheckBox class. It is used to enable or disable an option (false). When you click on a CheckBox, its status changes from “on” to “off” or from “off” to “on”. It derives from the JToggleButton class.
Constructors:
JCheckBox()
JCheckBox(String s)
JCheckBox(String text, boolean selected)
JCheckBox(Action a)
Java JRadioButton:
To make a radio button, use the JRadioButton class. It is used to select one of several possibilities. It is frequently used in exam systems or quizzes.
It should be added to ButtonGroup to allow only one radio button to be selected.
Constructors:
JRadioButton()
JRadioButton(String s)
JRadioButton(String s, boolean selected)
Java JComboBox:
The object of the Choice class is used to display a popup menu of options. A user-selected option is displayed at the top of a menu.
Constructors:
JComboBox()
JComboBox(Object[] items)
JComboBox(Vector<?> items)
Java JLabel:
Swingtoolkit in Java includes a JLabel Class. It is found in the javax.swing.JLabel class package. It is used to put text in a box. Only single-line text is permitted, and it cannot be modified directly.
Constructors:
JLabel()
JLabel(Icon i)
JLabel(String s)
JLabel(String s, Icon i, int horizontalAlignment)
Java JTextArea:
The Swing toolkit in Java includes a JTextArea Class. It is found in the javax.swing.JTextArea package. It is used to display text that spans numerous lines.
Constructors:
JTextArea()
JTextArea(String s)
JTextArea(int row, int column)
JTextArea(String s, int row, int column)
Example of a form:
Below given example incorporates the use of JButton, JRadioButton, JTextArea, JTextField, JLabel, MigLayout, ActionLIstener.
package gui1;
import java.awt.EventQueue;
import javax.swing.JFrame;
import javax.swing.JOptionPane;
import net.miginfocom.swing.MigLayout;
import javax.swing.JButton;
import java.awt.event.ActionListener;
import java.awt.event.ActionEvent;
import javax.swing.JLabel;
import javax.swing.JTextField;
import javax.swing.JCheckBox;
import javax.swing.JRadioButton;
import javax.swing.JTextArea;
public class testswing {
private JFrame frame;
private JTextField textField;
private JTextField textField_1;
/**
* Launch the application.
*/
public static void main(String[] args) {
EventQueue.invokeLater(new Runnable() {
public void run() {
try {
testswing window = new testswing();
window.frame.setVisible(true);
} catch (Exception e) {
e.printStackTrace();
}
}
});
}
/**
* Create the application.
*/
public testswing() {
initialize();
}
/**
* Initialize the contents of the frame.
*/
private void initialize() {
frame = new JFrame("Button Example");
frame.setBounds(100, 100, 450, 300);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.getContentPane().setLayout(new MigLayout("", "[][][][grow][][][][]", "[][][][][][][][grow][]"));
JLabel lblNewLabel = new JLabel("Name");
frame.getContentPane().add(lblNewLabel, "cell 1 1");
textField = new JTextField();
frame.getContentPane().add(textField, "cell 3 1,growx");
textField.setColumns(10);
JLabel lblNewLabel_1 = new JLabel("Email");
frame.getContentPane().add(lblNewLabel_1, "cell 1 2");
textField_1 = new JTextField();
frame.getContentPane().add(textField_1, "cell 3 2,growx");
textField_1.setColumns(10);
JLabel lblNewLabel_3 = new JLabel("Gender");
frame.getContentPane().add(lblNewLabel_3, "cell 1 3");
JRadioButton rdbtnNewRadioButton_1 = new JRadioButton("Female");
frame.getContentPane().add(rdbtnNewRadioButton_1, "cell 3 3");
JRadioButton rdbtnNewRadioButton = new JRadioButton("Male");
frame.getContentPane().add(rdbtnNewRadioButton, "cell 3 4");
JRadioButton rdbtnNewRadioButton_2 = new JRadioButton("TransGender");
frame.getContentPane().add(rdbtnNewRadioButton_2, "cell 3 5");
JLabel lblNewLabel_4 = new JLabel("Address");
frame.getContentPane().add(lblNewLabel_4, "cell 1 6");
JButton btnClickMe = new JButton("Save");
btnClickMe.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
JOptionPane.showMessageDialog(null, "Details saved");
}
});
JTextArea textArea = new JTextArea();
frame.getContentPane().add(textArea, "cell 3 6,grow");
frame.getContentPane().add(btnClickMe, "cell 3 8");
}
}
Output:
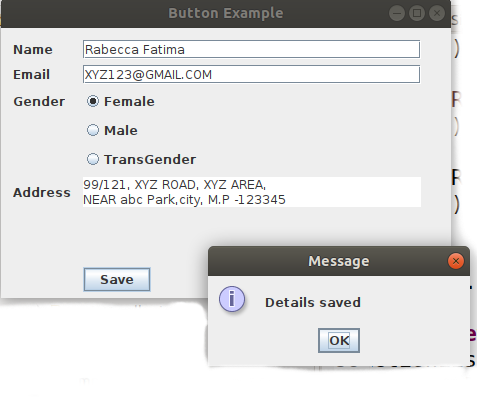
Note: also read about the Swing Class in Java
Follow Me
If you like my post, please follow me to read my latest post on programming and technology.
https://www.instagram.com/coderz.py/
https://www.facebook.com/coderz.py
Staying up to the mark is what defines me. Hi all! I’m Rabecca Fatima a keen learner, great enthusiast, ready to take new challenges as stepping stones towards flying colors.
Leave a Comment
You must be logged in to post a comment.