
Java AWT TextArea:
A TextArea class’s object is a multiline zone that displays text. It allows you to modify many lines of text at once. It derives from the TextComponent class.
We can type as much text as we wish in the text area. When the text in the text field exceeds the viewing area, the scroll bar appears, allowing us to scroll the text up and down, or right and left.
constructors:
TextArea() //It creates a new, empty text space with no content.
TextArea (int row, int column) //It creates a new text area with the number of rows and columns supplied and an empty string as text.
TextArea (String text) //It creates a new text area and inserts the provided text into it.
TextArea (String text, int row, int column) //It constructs a new text area with the specified text in the text area and specified number of rows and columns.
TextArea (String text, int row, int column, int scrollbars) //It creates a new text area with the supplied text in the text area, the specified number of rows and columns, and the specified visibility.
Java AWT Checkbox:
To build a checkbox, utilize the Checkbox class. It is used to enable or disable an option (false). When you click on a Checkbox, its status changes from “on” to “off” or from “off” to “on.”
Constructors:
Checkbox()
Checkbox(String label)
Checkbox(String label, boolean state)
Checkbox(String label, boolean state, CheckboxGroup group)
Checkbox(String label, CheckboxGroup group, boolean state)
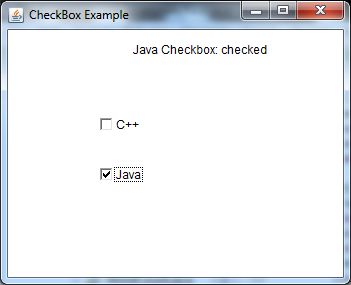
Java AWT CheckboxGroup:
The CheckboxGroup class object is used to group a set of Checkboxes. Only one check box button may be in the “on” state at any given time, with the remaining checkbox buttons in the “off” state. It derives from the object class.
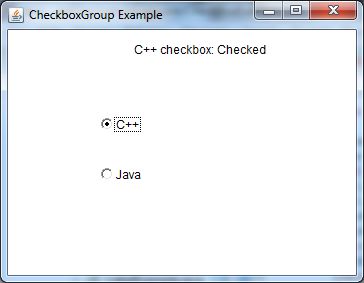
Java AWT Choice:
The object of the Choice class is used to display a popup menu of options. A user-selected option is displayed at the top of a menu. It derives from the Component class.
Constructor:
Choice() //It constructs a new choice menu.
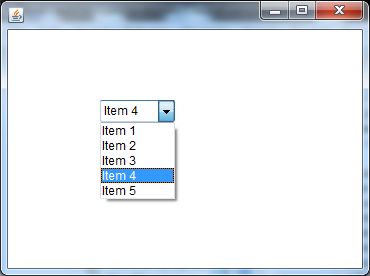
Java AWT List:
The List class object represents a list of text objects. The List class allows the user to select either one or numerous items. It derives from the Component class.
Constructors:
List() //It constructs a new scrolling list.
List(int row_num) //It creates a new scrolling list with the specified number of visible rows.
List(int row_num, Boolean multipleMode) //It creates a new scrolling list that displays the specified number of rows.
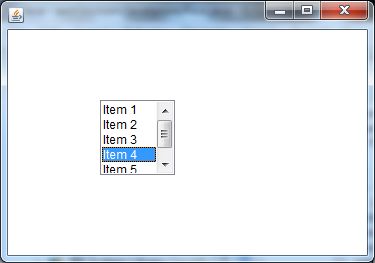
Java AWT MenuItem and Menu:
The MenuItem class object adds a simple labeled menu item to the menu. The items in a menu must be of the MenuItem class or one of its subclasses.
The Menu class’s object is a pull-down menu component that appears on the menu bar. It derives from the MenuItem class.

Java AWT Panel:
The Panel is the most basic container class. It gives a location for an application to attach any other component. It derives from the Container class.
It lacks a title bar.
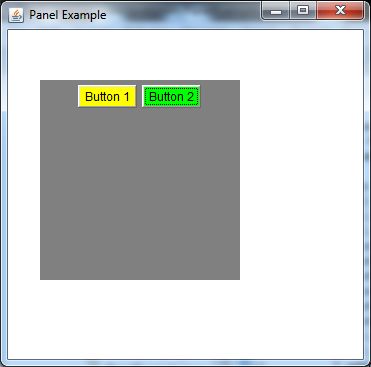
Java AWT Toolkit:
The Abstract Window Toolkit’s Toolkit class is the abstract superclass of all implementations. Toolkit subclasses are used to bind numerous components. It derives from the Object class.
Example:
We shall see an example of a form using Java Swing GUI Framework
import java.awt.BorderLayout;
import java.awt.EventQueue;
import javax.swing.JFrame;
import javax.swing.JPanel;
import javax.swing.border.EmptyBorder;
import net.miginfocom.swing.MigLayout;
import javax.swing.JLabel;
import javax.swing.JOptionPane;
import java.awt.Font;
import javax.swing.JTextField;
import javax.swing.JButton;
import java.awt.Color;
import java.awt.SystemColor;
import java.awt.event.ActionListener;
import java.awt.event.ActionEvent;
import java.awt.event.ComponentAdapter;
import java.awt.event.ComponentEvent;
public class Form extends JFrame {
private JPanel contentPane;
private JTextField textField;
private JTextField textField_1;
private JTextField textField_2;
private JTextField textField_3;
private JTextField textField_4;
private JTextField textField_5;
/**
* Launch the application.
*/
public static void main(String[] args) {
EventQueue.invokeLater(new Runnable() {
public void run() {
try {
Form frame = new Form();
frame.setVisible(true);
} catch (Exception e) {
e.printStackTrace();
}
}
});
}
/**
* Create the frame.
*/
public Form() {
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setBounds(100, 100, 450, 300);
contentPane = new JPanel();
contentPane.setBorder(new EmptyBorder(5, 5, 5, 5));
setContentPane(contentPane);
contentPane.setLayout(new MigLayout("", "[][][grow]", "[][][][][][][][][]"));
JLabel lblNewLabel = new JLabel("NAME");
lblNewLabel.setFont(new Font("Tahoma", Font.BOLD, 13));
contentPane.add(lblNewLabel, "cell 0 0,alignx center");
textField = new JTextField();
contentPane.add(textField, "cell 2 0,growx");
textField.setColumns(10);
JLabel lblNewLabel_4 = new JLabel("COURSE");
lblNewLabel_4.setFont(new Font("Tahoma", Font.BOLD, 13));
contentPane.add(lblNewLabel_4, "cell 0 1");
textField_3 = new JTextField();
contentPane.add(textField_3, "cell 2 1,growx");
textField_3.setColumns(10);
JLabel lblNewLabel_1 = new JLabel("ENROLL.No");
lblNewLabel_1.setFont(new Font("Tahoma", Font.BOLD, 13));
contentPane.add(lblNewLabel_1, "cell 0 2,alignx center");
textField_1 = new JTextField();
contentPane.add(textField_1, "cell 2 2,growx");
textField_1.setColumns(10);
JLabel lblNewLabel_5 = new JLabel("BRANCH");
lblNewLabel_5.setFont(new Font("Tahoma", Font.BOLD, 13));
contentPane.add(lblNewLabel_5, "cell 0 3,alignx center");
textField_4 = new JTextField();
contentPane.add(textField_4, "cell 2 3,growx");
textField_4.setColumns(10);
JLabel lblNewLabel_2 = new JLabel("YEAR");
lblNewLabel_2.setFont(new Font("Tahoma", Font.BOLD, 13));
contentPane.add(lblNewLabel_2, "cell 0 4,alignx center");
textField_2 = new JTextField();
contentPane.add(textField_2, "cell 2 4,growx");
textField_2.setColumns(10);
JLabel lblNewLabel_3 = new JLabel("SEMESTER");
lblNewLabel_3.setFont(new Font("Tahoma", Font.BOLD, 13));
contentPane.add(lblNewLabel_3, "cell 0 5,alignx center");
textField_5 = new JTextField();
contentPane.add(textField_5, "cell 2 5,growx");
textField_5.setColumns(10);
JButton btnNewButton = new JButton("SUBMIT");
btnNewButton.addComponentListener(new ComponentAdapter() {
@Override
public void componentShown(ComponentEvent e) {
}
});
btnNewButton.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
JOptionPane.showMessageDialog(null, "details submitted");
form2 ob =new form2();
ob.setVisible(true);
}
});
btnNewButton.setBackground(SystemColor.inactiveCaption);
btnNewButton.setForeground(Color.BLACK);
btnNewButton.setFont(new Font("Trebuchet MS", Font.BOLD, 13));
contentPane.add(btnNewButton, "cell 2 8,alignx center");
formhandler fm=new formhandler(textField,textField_1);
}
}
class formhandler implements ActionListener {
private JTextField ta,ts;
public void actionPerformed(ActionEvent e)
{
String name= ta.getText();
ts.setText(name);
}
public formhandler(JTextField ta,JTextField ts)
{
this.ta=ta;
this.ts=ts;
}
}
Output:
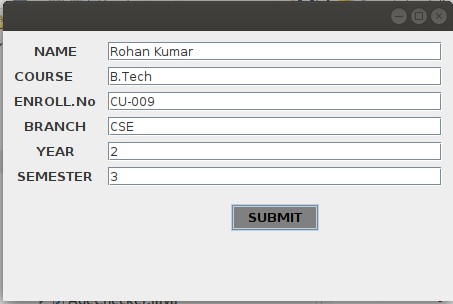
After filling out the details, click on submit and another window will pop up.
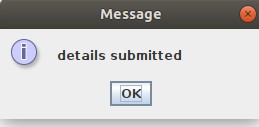
We shall see the working of all the components mentioned above in another example.
Output:
Note: also read about the JApplet class in Applet
Follow Me
If you like my post, please follow me to read my latest post on programming and technology.
https://www.instagram.com/coderz.py/
https://www.facebook.com/coderz.py
Staying up to the mark is what defines me. Hi all! I’m Rabecca Fatima a keen learner, great enthusiast, ready to take new challenges as stepping stones towards flying colors.
Leave a Comment
You must be logged in to post a comment.