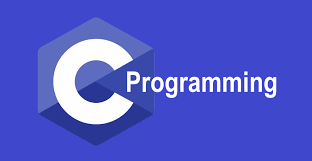
Data types in C refer to how much memory it occupies in storage and how the bit pattern stored is interpreted. To thoroughly define a variable, one needs to mention not only its type but also its storage class.
There are the following types of data types in C:
- Basic Types: They are arithmetic types and are further classified into (a) integer types and (b) floating-point types. For instance, int, char, float, double.
- Type void: The type specifier void indicates that no value is available.
- Derived types: They include (a) Pointer types, (b) Array types, (c) Structure types, (d) Union types and (e) Function types.
- Enumeration Data Type: They are again arithmetic types and they are used to define variables that can only assign certain discrete integer values throughout the program.i.e., enum.
Different data types also have different ranges up to which they can store numbers. These ranges may vary from compiler to compiler. That is to say, for a 16-bit compiler and a 32-bit compiler the range of the data types may vary. Here a 16-bit compiler means that when it compiles a C program it generates machine language code that is targeted towards working on a 16-bit microprocessor like Intel 8086/8088. As against this, a 32-bit compiler like VC++ generates machine language code that is targeted towards a 32-bit microprocessor like Intel Pentium.
List of all data types in C:
Data Types | Memory Size | Range | Format |
---|---|---|---|
char | 1 byte | −128 to 127 | %c |
signed char | 1 byte | −128 to 127 | %c |
unsigned char | 1 byte | 0 to 255 | %c |
short | 2 byte | −32,768 to 32,767 | %d |
signed short | 2 byte | −32,768 to 32,767 | %d |
unsigned short | 2 byte | 0 to 65,535 | %u |
int | 2 byte | −32,768 to 32,767 | %d |
signed int | 2 byte | −32,768 to 32,767 | %d |
unsigned int | 2 byte | 0 to 65,535 | %u |
short int | 2 byte | −32,768 to 32,767 | %d |
signed short int | 2 byte | −32,768 to 32,767 | %d |
unsigned short int | 2 byte | 0 to 65,535 | %u |
long int | 4 byte | -2,147,483,648 to 2,147,483,647 | %ld |
signed long int | 4 byte | -2,147,483,648 to 2,147,483,647 | %ld |
unsigned long int | 4 byte | 0 to 4,294,967,295 | %lu |
float | 4 byte | %f | |
double | 8 byte | %lf | |
long double | 10 byte | %Lf |
sizeof() operator:
The sizeof() operator in C is used to check the size of a variable.
example:
#include <stdio.h>
int main()
{
int a = 30;
char b = '!';
double c = 8.9;
// printing the variables defined
// above along with their sizes
printf("Size of char: %d",sizeof(char));
printf("Size of int: %d",sizeof(int));
printf("Size of double:%d",sizeof(double));
return 0;
}
output:
Size of char:1
Size of int: 2
Size of double:8
note: also read about Keywords in C & Variables & Constants in C.
Follow Me
If you like my post please follow me to read my latest post on programming and technology.
https://www.instagram.com/coderz.py/
https://www.facebook.com/coderz.py
Staying up to the mark is what defines me. Hi all! I’m Rabecca Fatima a keen learner, great enthusiast, ready to take new challenges as stepping stones towards flying colors.
Leave a Comment
You must be logged in to post a comment.