
What is an Exception?
An exception is a problem that arises during the execution of a program. A C++ exception is a response to an exceptional circumstance that arises while a program is running
What is Exception Handling?
In C++, exception handling is the process of dealing with runtime errors. We handle exceptions so that the application’s normal flow can be maintained even when runtime errors occur.
The exceptions can be broadly categorized into two types:
- Compile Time
- Run Time
Exception handling in C++ is based on three keywords: try, catch, and throw.
try block:
A try block identifies a section of code for which specific exceptions will be raised. It is immediately followed by one or more catch blocks.
catch block:
A program catches an exception with an exception handler at the point in the program where the problem should be handled. The catch block is designed to catch errors and handle exceptions. Multiple catch blocks can be used to handle different types of exceptions and perform different actions when they occur.
throw:
When a problem occurs, a program throws an exception. This is accomplished with the throw keyword. A throw expression takes one parameter, which is passed to the handler.
Syntax:
try
{
//code
throw parameter;
}
catch(exceptionname ex)
{
//code to handle exception
}
Why Exception Handling?
Exception handling has the following major advantages over traditional error handling:
- Error Handling code separated from Normal Code Functions/Methods
- Can handle only the exceptions they choose
- Classification of Error Types
Example: code without exception handling
#include <iostream>
using namespace std;
double division(int a, int b) {
return (a/b);
}
int main () {
int x = 50;
int y = 0;
double z = 0;
z = division(x, y);
cout << z << endl;
return 0;
}
Output:
The above program will not run and will show a runtime error on the screen because we are trying to divide a number with 0, which is not possible.
Example: code with exception handling
#include <iostream>
using namespace std;
double division(int a, int b) {
if( b == 0 ) {
throw "Division by zero condition!";
}
return (a/b);
}
int main () {
int x = 50;
int y = 0;
double z = 0;
try {
z = division(x, y);
cout << z << endl;
} catch (const char* msg) {
cerr << msg << endl;
}
return 0;
}
Output:
Division by zero condition!
Using Multiple catch blocks:
In a program, we can use multiple catch blocks to handle different types of exceptions in different ways.
Example:
#include<iostream>
using namespace std;
int main()
{
int a=10, b=0, c;
try
{
//if a is divided by b(which has a value 0);
if(b==0)
throw(c);
else
c=a/b;
}
catch(char c) //catch block to handle/catch exception
{
cout<<"Caught exception : char type ";
}
catch(int i) //catch block to handle/catch exception
{
cout<<"Caught exception : int type ";
}
catch(short s) //catch block to handle/catch exception
{
cout<<"Caught exception : short type ";
}
cout<<"\n end of execution";
}
Output:
Caught exception : int type
end of execution
Standard Exceptions in C++:
C++ comes with a set of standard exceptions defined that we can use in our programs
Exception | Description |
---|---|
std::exception | An exception and parent class of all the standard C++ exceptions. |
std::bad_alloc | This can be thrown by new. |
std::bad_cast | This can be thrown by dynamic_cast. |
std::bad_exception | This is useful to handle unexpected exceptions in a C++ program. |
std::bad_typeid | This can be thrown by typeid. |
std::logic_error | An exception that theoretically can be detected by reading the code. |
std::domain_error | This is an exception thrown when a mathematically invalid domain is used. |
std::invalid_argument | This is thrown due to invalid arguments. |
std::length_error | This is thrown when a too-big std::string is created. |
std::out_of_range | This can be thrown by the ‘at’ method, for example, a std::vector and std::bitset<>::operator[](). |
std::runtime_error | An exception that theoretically cannot be detected by reading the code. |
std::overflow_error | thrown if a mathematical overflow occurs. |
std::range_error | This occurs when you try to store a value that is out of range. |
std::underflow_error | This is thrown if a mathematical underflow occurs. |
parent-child class hierarchy of exceptions:
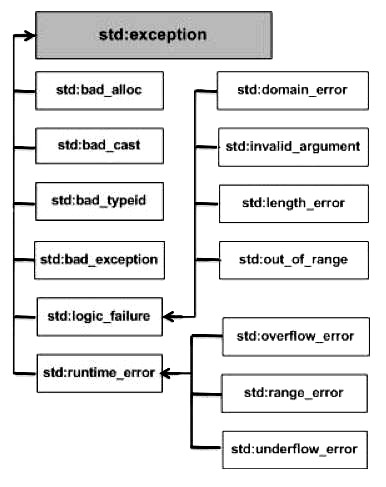
Note: also read about Operator Overloading in C++
Follow Me
Please follow me to read my latest post on programming and technology if you like my post.
https://www.instagram.com/coderz.py/
https://www.facebook.com/coderz.py
Staying up to the mark is what defines me. Hi all! I’m Rabecca Fatima a keen learner, great enthusiast, ready to take new challenges as stepping stones towards flying colors.
Leave a Comment
You must be logged in to post a comment.