
With its I/O package, Java includes a variety of Streams that make it easier for the user to carry out any input-output tasks. For complete I/O operation execution, these streams support all object, data-type, character, and file kinds.
Stream:
A data stream is a collection of data. A stream in Java is made up of bytes. It resembles a flowing stream of water, which is why it is called a stream.
Before looking at different input and output streams, let’s take a closer look at the three standard or default streams that Java offers and are also the most widely used:
- System.in: The standard input stream, known as system.in, reads characters from the keyboard and other standard input devices.
- System.out: It is the standard output stream used to display a program’s output on an output device, such as a computer screen.
- System.err: This is the standard error stream used to print any standard output device or a computer screen with any error information that a program might produce.
Under the java.io package, Stream is contained by Java. Java specifies two different stream types. It’s them,
- Byte stream: A convenient method for processing byte input and output is the byte stream.
- Character Stream: It offers a practical method for managing character input and output. Character stream can be internationalized because it uses Unicode.
Java Byte Stream Class:
The two abstract classes InputStream and OutputStream at the top of the hierarchy are used to define bytes streams.
OutputStream:
An output stream is used by a Java application to write data to a destination, such as a file, an array, a peripheral device, or a socket.
Useful methods of OutputStream:
- public void write(int)throws IOException– write a byte to the active output stream using this method.
- public void write(byte[])throws IOException– is used to write an array of bytes to the current output stream.
- public void flush()throws IOException– flushes the current output stream.
- public void close()throws IOException- is used to close the current output stream.
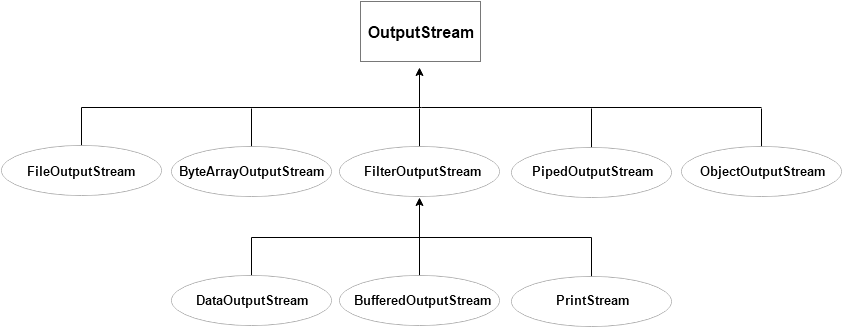
InputStream:
A Java application utilizes an input stream when reading data from a source—a file, an array, a peripheral device, or a socket.
methods of InputStream:
- public abstract int read()throws IOException- scans the incoming data stream’s subsequent byte. At the conclusion of the file, it returns 1.
- public int available()throws IOException– returns an estimate of the number of bytes that can be read from the current input stream.
- public void close()throws IOException– is used to close the current input stream.
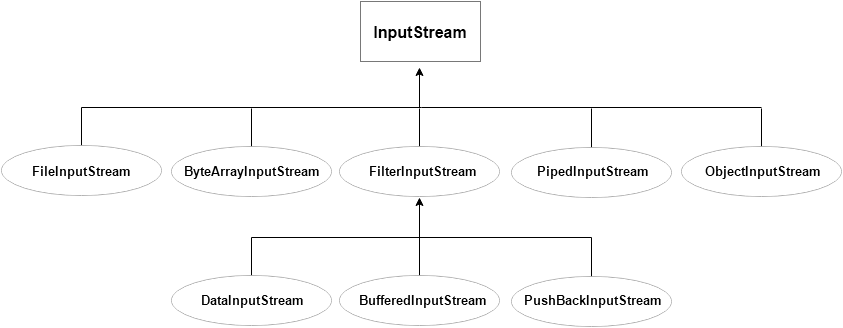
Example:
// Java Program illustrating the
// Byte Stream to copy
// contents of one file to another file.
import java.io.*;
public class Coderz {
public static void main(
String[] args) throws IOException
{
FileInputStream sourceStream = null;
FileOutputStream targetStream = null;
try {
sourceStream
= new FileInputStream("sorcefile.txt");
targetStream
= new FileOutputStream("targetfile.txt");
// Reading source file and writing
// content to target file byte by byte
int temp;
while ((
temp = sourceStream.read())
!= -1)
targetStream.write((byte)temp);
}
finally {
if (sourceStream != null)
sourceStream.close();
if (targetStream != null)
targetStream.close();
}
}
}
Output:
Shows contents of file test.txt
Java Character Stream Class:
Reader and Writer are the two abstract classes at the top of the hierarchy used to define character streams.
Stream class | Description |
---|---|
BufferedReader | It is used to handle buffered input stream. |
FileReader | This is an input stream that reads from a file. |
InputStreamReader | This input stream is used to translate byte to character. |
OutputStreamReader | This output stream is used to translate character to byte. |
Reader | This is an abstract class that defines character stream input. |
PrintWriter | This contains the most used print() and println() method |
Writer | This is an abstract class that defines character stream output. |
BufferedWriter | This is used to handle the buffered output stream. |
FileWriter | This is used to output stream that writes to the file. |
Example:
import java. Io *;
// Java Program illustrating that
// we can read a file in a human-readable
// format using FileReader
class Coderz
{
public static void main(String[] args)
{
try
{
File fl = new File("d:/myfile.txt");
// Reading sourcefile and
// writing content to target file
// character by character.
BufferedReader br = new BufferedReader(new FileReader(fl)) ;
String str;
while ((str=br.readLine())!=null)
{
System.out.println(str);
}
br.close();
fl.close();
// Closing stream as no longer in use
}
catch(IOException e) {
e.printStackTrace();
}
}
}
Output:
Shows contents of file
Note: also read about the Autoboxing and Unboxing in Java
Follow Me
If you like my post, please follow me to read my latest post on programming and technology.
https://www.instagram.com/coderz.py/
https://www.facebook.com/coderz.py
Staying up to the mark is what defines me. Hi all! I’m Rabecca Fatima a keen learner, great enthusiast, ready to take new challenges as stepping stones towards flying colors.
Leave a Comment
You must be logged in to post a comment.