
JApplet is a public java swing class created for developers often developed in Java. JApplet is usually written in Java byte code and executed using a Java virtual machine (JVM) or Applet reader from Sun Microsystems. It was initially released in 1995.
JApplet can be developed in any programming language and then compiled into Java byte code.
Example: EventJApplet.class
import java.applet.*;
import javax.swing.*;
import java.awt.event.*;
public class EventJApplet extends JApplet implements ActionListener{
JButton b;
JTextField tf;
public void init(){
tf=new JTextField();
tf.setBounds(30,40,150,20);
b=new JButton("Click");
b.setBounds(80,150,70,40);
add(b);add(tf);
b.addActionListener(this);
setLayout(null);
}
public void actionPerformed(ActionEvent e){
tf.setText("Welcome to Coderz");
}
}
JApplet.html
<html>
<body>
<applet code="EventJApplet.class" width="300" height="300">
</applet>
</body>
</html>
Output:
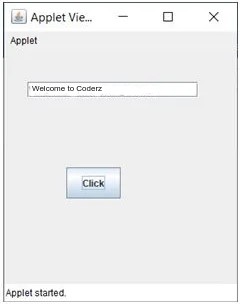
Applet Painting:
The mouseDragged() method of MouseMotionListener allows us to paint in the applet.
Example: MouseDrag.class
import java.awt.*;
import java.awt.event.*;
import java.applet.*;
public class MouseDrag extends Applet implements MouseMotionListener{
public void init(){
addMouseMotionListener(this);
setBackground(Color.white);
}
public void mouseDragged(MouseEvent me){
Graphics g=getGraphics();
g.setColor(Color.blue);
g.fillOval(me.getX(),me.getY(),6,6);
}
public void mouseMoved(MouseEvent me){}
}
MouseDrag.html
<html>
<body>
<applet code="MouseDrag.class" width="300" height="300">
</applet>
</body>
</html>
Output:
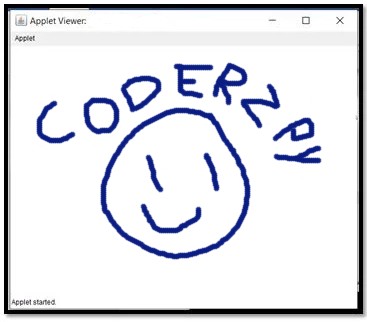
Digital Clock in Applet:
The Calendar and SimpleDateFormat classes can be used to make a digital clock. Consider the following simple example:
DigitalClock.class:
import java.applet.*;
import java.awt.*;
import java.util.*;
import java.text.*;
public class DigitalClock extends Applet implements Runnable {
Thread t = null;
int hours=0, minutes=0, seconds=0;
String timeString = "";
public void init() {
setBackground( Color.Black);
}
public void start() {
t = new Thread( this );
t.start();
}
public void run() {
try {
while (true) {
Calendar cal = Calendar.getInstance();
hours = cal.get( Calendar.HOUR_OF_DAY );
if ( hours > 12 ) hours -= 12;
minutes = cal.get( Calendar.MINUTE );
seconds = cal.get( Calendar.SECOND );
SimpleDateFormat formatter = new SimpleDateFormat("hh:mm:ss");
Date date = cal.getTime();
timeString = formatter.format( date );
repaint();
t.sleep( 1000 ); // interval given in milliseconds
}
}
catch (Exception e) { }
}
public void paint( Graphics g ) {
g.setColor( Color.white );
g.drawString( timeString, 50, 50 );
}
}
DigitalClock.html
<html>
<body>
<applet code="DigitalClock.class" width="300" height="300">
</applet>
</body>
</html>
Note: also read about the Graphics in Applet
Follow Me
If you like my post, please follow me to read my latest post on programming and technology.
https://www.instagram.com/coderz.py/
https://www.facebook.com/coderz.py
Staying up to the mark is what defines me. Hi all! I’m Rabecca Fatima a keen learner, great enthusiast, ready to take new challenges as stepping stones towards flying colors.
Leave a Comment
You must be logged in to post a comment.