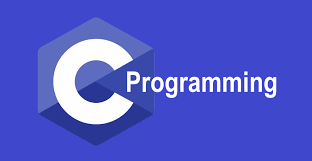
C allows us to nest one structure within another structure, which allows us to design complicated data types. This type of structure is known as nested structure.
For example, we may need to store the result of an entity student in a structure. The attribute result may also have the subparts like subject name, marks, and status. Hence, to store the result of the student, we need to store the result of the student into a separate structure and nest the structure result into the structure student.
Example of nested structure:
#include<stdio.h>
struct result
{
char sub_name[20];
float marks;
};
struct student
{
char name[20];
int roll;
char class[14];
struct result res;
};
void main ()
{
struct student stu;
printf("Enter student information?\n");
scanf("%s %s %d %f",stu.name,stu.res.sub_name, &stu.roll, &stu.res.marks);
printf("Printing the student information....\n");
printf("Name: %s Subject: %s Roll: %d Marks: %f",stu.name,stu.res.sub_name, stu.roll, stu.res.marks);
}
Output:
Enter student information?
coderzpy maths 21 99
Printing the student information....
Name: coderzpy Subject: maths Roll: 21 Marks: 99.000000
The structure can be nested as shown below.
- Independent structure
- Embedded structure
Independent structure:
Here, we establish two structures, however, the dependent structure should be used as a member of the primary structure. For instance,
struct Date
{
int dd;
int mm;
int yyyy;
};
struct Employee
{
int id;
char name[20];
struct Date doj;
}emp1;
Embedded structure:
We can declare the structure within the structure using the embedded structure. As a result, it takes fewer lines of code but cannot be utilized in numerous data structures. For instance,
struct Employee
{
int id;
char name[20];
struct Date
{
int dd;
int mm;
int yyyy;
}doj;
}emp1;
Note: also read about the Structure in C& Array of Structure
Follow Me
If you like my post please follow me to read my latest post on programming and technology.
https://www.instagram.com/coderz.py/
https://www.facebook.com/coderz.py
Staying up to the mark is what defines me. Hi all! I’m Rabecca Fatima a keen learner, great enthusiast, ready to take new challenges as stepping stones towards flying colors.
Leave a Comment