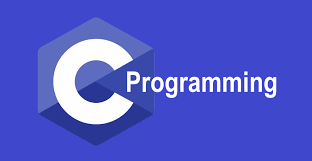
Operators in C are symbols that are used to perform operations on operands(operands can be a constant, a variable, or a function on which operation is performed using the operators ). There can be many types of operations like arithmetic, logical, bitwise, etc.
The following types of operators are supported in the C language −
- Arithmetic Operators
- Relational Operators
- Logical Operators
- Bitwise Operators
- Assignment Operators
- Misc Operator
Arithmetic operators:
These operators are used to perform various arithmetic operations such as add, subtract, modulus, etc.
For instance, variable a holds 40 and variable b holds 20 then −
Operator | Description | Example |
++ | Unary operator incrementing operand by 1 | ++a = 41 |
— | Unary operator decrementing operand by 1 | –a = 19 |
+ | Adds two operands | a+b = 60 |
– | Subtract two operands | a-b = 20 |
* | Multiply two operands | a*b = 800 |
/ | Divides numerator by de-numerator | a/b = 2 |
% | Modulus Operator used to calculate the remainder after division | b%a = 20 |
Relational Operators:
Relational operators in C are also known as comparison operators. Assume variable a holds’ 40′ and variable b holds ’20’ then −
Operator | Description | Example |
---|---|---|
== | if the values of two operands are equal then returns true otherwise false. | (a == b) is false. |
!= | if the values of two operands are not equal then returns true otherwise false. | (a != b) is true. |
> | if the value of left operand is greater than the value of right operand then returns true otherwise false. | (a > b) is true. |
< | if the value of left operand is less than the value of right operand then returns true otherwise false. | (a < b) is false. |
>= | if the value of left operand is greater than or equal to the value of right operand then returns true otherwise false. | (a >= b) is true. |
<= | if the value of left operand is less than or equal to the value of right operand then returns true otherwise false. | (a <= b) is false. |
Logical Operators:
A logical operator in C is a symbol or word used to connect two or more expressions such that the value of the compound expression produced depends only on that of the original expressions and on the meaning of the operator.
Operator | Description | Example |
---|---|---|
&& | Logical AND operator. If both the operands are non-zero, then returns true otherwise false. | (a && b) is false. |
|| | Logical OR Operator. If any of the two operands is non-zero, then returns true otherwise false. | (a || b) is true. |
! | Logical NOT Operator. It is used to reverse the logical state of its operand. If a condition is true, then the Logical NOT operator will make it false. | !(a && b) is true. |
Bitwise Operators:
This operator works on bits and performs bit-by-bit operations. The truth tables for &, |, and ^ is as follows −
a | b | a & b | a | b | a ^ b |
---|---|---|---|---|
0 | 0 | 0 | 0 | 0 |
0 | 1 | 0 | 1 | 1 |
1 | 1 | 1 | 1 | 0 |
1 | 0 | 0 | 1 | 1 |
Assume variable ‘a’ holds 60 and variable ‘b’ holds 13, then −
Operator | Description | Example |
---|---|---|
& | Binary AND Operator returns 1 if both bits are 1 otherwise returns 0. | (A & B) = 12, i.e., 0000 1100 |
| | Binary OR Operator returns 1 if either bit is 1 otherwise 0. | (A | B) = 61, i.e., 0011 1101 |
^ | Binary XOR Operator returns 1 if one of the bits is 1 otherwise 0. | (A ^ B) = 49, i.e., 0011 0001 |
~ | Binary One’s Complement Operator is unary and has the effect of ‘flipping’ bits. | (~A ) = ~(60), i.e,. -0111101 |
<< | Binary Left Shift Operator. The left operands value is moved left by the number of bits specified by the right operand. | A << 2 = 240 i.e., 1111 0000 |
>> | Binary Right Shift Operator. The left operands value is moved right by the number of bits specified by the right operand. | A >> 2 = 15 i.e., 0000 1111 |
Assignment Operators
The following table lists the assignment operators supported by the C language −
Operator | Description | Example |
---|---|---|
= | Simple assignment operator. Assigns values from right side operands to left side operand | C = A + B will assign the value of A + B to C |
+= | Add AND assignment operator. It adds the right operand to the left operand and assigns the result to the left operand. | C += A is equivalent to C = C + A |
-= | Subtract AND assignment operator. It subtracts the right operand from the left operand and assigns the result to the left operand. | C -= A is equivalent to C = C – A |
*= | Multiply AND assignment operator. It multiplies the right operand with the left operand and assigns the result to the left operand. | C *= A is equivalent to C = C * A |
/= | Divide AND assignment operator. It divides the left operand with the right operand and assigns the result to the left operand. | C /= A is equivalent to C = C / A |
%= | Modulus AND assignment operator. It takes modulus using two operands and assigns the result to the left operand. | C %= A is equivalent to C = C % A |
<<= | Left shift AND assignment operator. | C <<= 2 is same as C = C << 2 |
>>= | Right shift AND assignment operator. | C >>= 2 is same as C = C >> 2 |
&= | Bitwise AND assignment operator. | C &= 2 is same as C = C & 2 |
^= | Bitwise exclusive OR and assignment operator. | C ^= 2 is same as C = C ^ 2 |
|= | Bitwise inclusive OR and assignment operator. | C |= 2 is same as C = C | 2 |
Misc Operators ↦ sizeof & ternary:
Besides the operators discussed above, there are a few other important operators including sizeof and ? : supported by the C Language.
Operator | Description | Example |
---|---|---|
sizeof() | Returns the size of a variable. | sizeof(a), where a is an integer, will return 4. |
& | Returns the address of a variable. | &a; returns the actual address of the variable. |
* | Pointer to a variable. | *a; |
? : | Conditional Expression. | If Condition is true ? then value X : Otherwise, value Y |
note: also read about Variables & Constants in C & Data Types in C.
Follow Me
If you like my post please follow me to read my latest post on programming and technology.
https://www.instagram.com/coderz.py/
https://www.facebook.com/coderz.py
Staying up to the mark is what defines me. Hi all! I’m Rabecca Fatima a keen learner, great enthusiast, ready to take new challenges as stepping stones towards flying colors.
Leave a Comment
You must be logged in to post a comment.