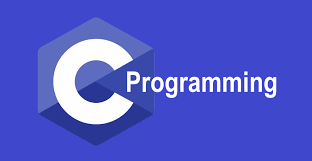
Typedef in C is used to redefine the name of the existing variable type. It generally increases the readability and convenience of complex data types.
Syntax:
typedef <name_of_data> <alias_name>;
For instance, consider the following statement in which the type unsigned long int is redefined to be of the type TWOWORDS:
unsigned long int var1, var2;
typedef unsigned long int TWOWORDS;
TWOWORDS var1,var2;
Thus, typedef in C provides a short and meaningful way to call a data type. Usually, uppercase letters are used to make it clear that we are dealing with a renamed data type
We can also use typedef with structures:
struct employee
{
char name[30];
int age;
float bs;
};
typedef struct employee EMP;
EMP e1, e2;
Example:
#include<stdio.h>
struct employee
{
char name[30];
int age;
float bs;
};
typedef struct employee P;
P e1, e2;
void main( )
{
printf("\nEnter Employee record:\n");
printf("\nEmployee name:\t");
scanf("%s", e1.name);
printf("\nEnter Employee salary: \t");
scanf("%d", &e1.age);
printf("\n name is %s", e1.name);
printf("\nsalary is %d", e1.age);
}
Output:
Enter Employee record:
Employee name: Coderzpy
Enter Employee salary: 190000
name is Coderzpy
salary is 190000
Thus, by reducing the length and clear complexness of data types, typedef can help to clarify source listing and save time and energy spent in understanding a program.
typedef can also be used to rename pointer data types as shown:
struct employee
{
char name[30];
int age;
float bs;
};
typedef struct employee * P;
P e1, e2;
e1->age =32;
Note: also read about the Nested Structure in C
Follow Me
If you like my post please follow me to read my latest post on programming and technology.
https://www.instagram.com/coderz.py/
https://www.facebook.com/coderz.py
Staying up to the mark is what defines me. Hi all! I’m Rabecca Fatima a keen learner, great enthusiast, ready to take new challenges as stepping stones towards flying colors.
Leave a Comment
You must be logged in to post a comment.