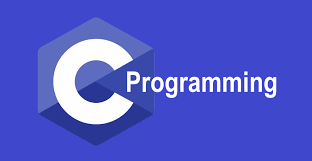
A union is a particular data type in C that allows several data types to be stored in the same memory address. A union can have numerous members, but only one member can have value at any given time. Unions are a convenient approach to use the same memory region for numerous purposes.
Both Structures and unions are used to group several variables. But while a structure enables us to treat a number of different variables stored at different places in memory, a union enables us to treat the same space in memory as a number of different variables. That is, a union offers a way for a section of memory to be treated as a variable of one type on one occasion, and as a different variable of a different type on another occasion.
Defining a union:
The union
keyword define unions.
For instance,
union car
{
char name[50];
int price;
};
Creating union variables
When a union is defined, a user-defined type is created. However, no memory is set aside. Variables must be created to allocate memory for a given union type and work with it.
union car
{
char name[50];
int price;
};
int main()
{
union car car1, car2, *car3;
return 0;
}
Members of a union can gain access.
We employ the ‘.‘ operator to gain access to union members The ‘->‘ operator is used to access pointer variables.
In the preceding example,
- Car1.price is used to get the price of car1.
- To get the price of a car3, use (*car3).price or car3->price.
Note: the size of a union variable will always be the size of its largest element.
Union of structure
Just as one structure can be nested within another, a union too can be nested in another union. There can be a union in a structure or a structure in a union.
Example:
// Online C compiler to run C program online
#include <stdio.h>
int main() {
struct a
{
int i;
char c[2];
};
struct b
{
int j;
char d[2];
};
union z
{
struct a key;
struct b data;
};
union z strange;
strange.key.i=512;
strange.data.d[0]=0;
strange.data.d[1]=32;
printf("\n%d",strange.key.i);
printf("\n%d",strange.data.j);
printf("\n%d",strange.key.c[0]);
printf("\n%d",strange.data.d[0]);
printf("\n%d",strange.key.c[1]);
printf("\n%d",strange.data.d[1]);
return 0;
}
Output:
512
512
0
0
32
32
here, we access the elements of the union in this program using the ‘.‘ operator twice. Thus,
strange.key.i
refers to the variable i in the structure key in the union strange.
Note: also read about the Typedef in C
Follow Me
If you like my post please follow me to read my latest post on programming and technology.
https://www.instagram.com/coderz.py/
https://www.facebook.com/coderz.py
Staying up to the mark is what defines me. Hi all! I’m Rabecca Fatima a keen learner, great enthusiast, ready to take new challenges as stepping stones towards flying colors.
Leave a Comment
You must be logged in to post a comment.