Strings are Objects in Java that are internally backed by a char array. They are immutable because arrays are immutable (they can’t grow). Every time you make a change to a String, a new String is created.
An array of characters functions similarly to a Java string. Consider the following scenario:
char[] ch={'c','o','d','e','r','z','p','y'};
String s=new String(ch);
is the same as:
String s="coderzpy";
The Serializable, Comparable, and CharSequence interfaces are implemented by the Java String class, as shown in the diagram below.
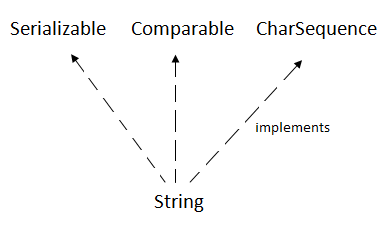
Interface for CharSequences:
To represent a sequence of characters, the CharSequence interface is used. It is implemented by the String, StringBuffer, and StringBuilder classes. This means that these three classes can be used to create strings in Java.
Memory allotment of String
A String Object will be created in the string constant pool whenever it is created as a literal. This allows JVM to optimize String literal initialization.
The string can also be dynamically allocated by using the new operator. Strings that are dynamically allocated are given a new memory location in the heap. The string constant pool will not include this string.
For instance:
String str = new String("Coderzy");
Example to declare String :
// Java code to illustrate String
import java.io.*;
import java.lang.*;
class Test {
public static void main(String[] args)
{
// Declare String without using new operator
String s = "student at";
// Prints the String.
System.out.println("String s = " + s);
// Declare String using new operator
String s1 = new String("Coderzpy");
// Prints the String.
System.out.println("String s1 = " + s1);
}
}
Output:
String s = student at
String s1 = Coderzpy
Note: also read about the Interface vs Abstract class
Follow Me
If you like my post, please follow me to read my latest post on programming and technology.
https://www.instagram.com/coderz.py/
https://www.facebook.com/coderz.py
Staying up to the mark is what defines me. Hi all! I’m Rabecca Fatima a keen learner, great enthusiast, ready to take new challenges as stepping stones towards flying colors.