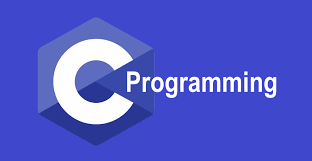
A palindrome is a sequence that, when reversed, looks exactly like the original. For example, abba, level, 232, and so on.
Let’s look at a program that uses the recursive technique to determine whether a number is a palindrome or not.
#include<stdio.h>
// declaring the recursive function
int isPalin(int );
int n;
int main()
{
int palindrome;
printf("\n\nEnter a number to check for Palindrome: ");
scanf("%d", &n);
palindrome = isPalin(n);
if(palindrome == 1)
printf("\n\n\n%d is palindrome\n\n", n);
else
printf("\n\n\n%d is not palindrome\n\n", n);
return 0;
}
int isPalin(int num)
{
static int sum = 0;
if(num != 0)
{
sum = sum *10 + num%10;
isPalin(num/10); // recursive call same as while(n!=0) using loop
}
else if(sum == n)
return 1;
else
return 0;
}
Output:
When the input is 121.
Enter a number to check for Palindrome: 121
121 is palindrome
When the input is 243.
Enter a number to check for Palindrome: 243
243 is not palindrome
Note: also read about the Command Line Argument
Follow Me
If you like my post please follow me to read my latest post on programming and technology.
https://www.instagram.com/coderz.py/
https://www.facebook.com/coderz.py
Staying up to the mark is what defines me. Hi all! I’m Rabecca Fatima a keen learner, great enthusiast, ready to take new challenges as stepping stones towards flying colors.
Leave a Comment
You must be logged in to post a comment.