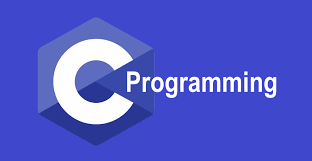
In C, a pointer is a variable that stores the address of another variable. This variable can be of any type, including int, char, array, function, or pointer. The pointer’s size is determined by the architecture. However, in 32-bit architecture, a pointer is 2 bytes in size.
Pointer Notation
Consider the declaration,
int a=53
int *i=&a;
This declaration tells the c compiler to:
- Reserve space in memory to hold the integer value.
- Associate the name with this memory location.
- Store the value 3 at this location.
- store the address of the variable in pointer i which is also provided with another memory location.
- With the help of * (indirection operator), we can print the value of pointer variable i.
- The address returned by the operator ‘&’ is the address of a variable. However, in order to display the address of a variable, we must use %u.
#include<stdio.h>
int main(){
int a=3;
int *i;
i=&a;//stores the address of number variable
printf("Address of i variable is %u \n",i); // i contains the address of the number therefore printing p gives the address of number.
printf("Value of i variable is %d \n",*i); // we will get the value stored at the address contained by i.
return 0;
}
Output:
Address of i variable is 571578428
Value of i variable is 3
The * is used to dereference a pointer.
We can create pointers for various data types. For instance,
Pointer to array:
int a[10];
int *p[10]=&a; // Variable p of type pointer is pointing to the address of an integer array a.
Pointer to function:
void show (int);
void(*p)(int) = &display; // Pointer p is pointing to the address of a function
Pointer to structure:
struct t {
int i;
float f;
}ref;
struct t *p = &ref;
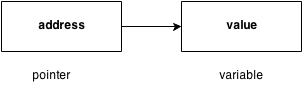
Use of a pointer in c:
In the C programming language, pointers can be used in a variety of ways.
1) Allocation of dynamic memory
We can use the pointer to dynamically allocate memory in C using the malloc() and calloc() functions.
2) Structures, Functions, and Arrays
Pointers are commonly used in arrays, functions, and structures in the C programming language. It shortens the code and boosts performance.
Note: also read about the Unions in C
Follow Me
If you like my post please follow me to read my latest post on programming and technology.
https://www.instagram.com/coderz.py/
https://www.facebook.com/coderz.py
Staying up to the mark is what defines me. Hi all! I’m Rabecca Fatima a keen learner, great enthusiast, ready to take new challenges as stepping stones towards flying colors.
Leave a Comment
You must be logged in to post a comment.