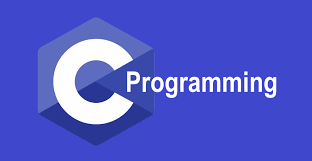
Just like any other data type, we can perform various arithmetic operations on pointers.
All pointers int, float, long, and double are 2 bytes on a 16-bit machine.
When we perform any arithmetic function, such as increment on a pointer, the size of their primitive data type changes.
Remember the following data type storage sizes in a 16 bit machine:
C Data types / storage Size | Range |
char / 1 | –127 to 127 |
int / 2 | –32,767 to 32,767 |
float / 4 | 1E–37 to 1E+37 with six digits of precision |
double / 8 | 1E–37 to 1E+37 with ten digits of precision |
long double / 10 | 1E–37 to 1E+37 with ten digits of precision |
long int / 4 | –2,147,483,647 to 2,147,483,647 |
short int / 2 | –32,767 to 32,767 |
unsigned short int / 2 | 0 to 65,535 |
signed short int / 2 | –32,767 to 32,767 |
long long int / 8 | –(2power(63) –1) to 2(power)63 –1 |
signed long int / 4 | –2,147,483,647 to 2,147,483,647 |
unsigned long int / 4 | 0 to 4,294,967,295 |
unsigned long long int / 8 | 2(power)64 –1 |
Pointer Arithmetic Examples:
int* a;
a++;
In the preceding example, the pointer will be two bytes long. When we increment it, it will increase by two bytes because int is also two bytes.
For instance:
#include<stdio.h>
int main()
{
int b;
int *p;
int **ptr;
b = 10;
/* take the address of b */
p = &b;
/* take the address of p using address of operator & */
ptr = &p;
/* take the value using ptr */
printf("Value of b = %d\n", b );
printf("Value available at *p = %d\n", *p );
printf("Value available at **ptr = %d\n", **ptr);
/* take the address */
printf("Value of &b = %u\n", &b );
printf("Value available at p = %u\n", p );
printf("Value available at ++p = %u\n", ++p );
printf("Value available at ptr = %u\n", ptr);
printf("Value available at ++ptr = %u\n", ++ptr);
return 0;
}
Output:
/tmp/L66gawGDLh.o
Value of b = 10
Value available at *p = 10
Value available at **ptr = 10
Value of &b = 1618367364
Value available at p = 1618367364
Value available at ++p = 1618367366
Value available at ptr = 1618367368
Value available at ++ptr = 1618367380
Here, we can clearly see that the value of ++p and ++ptr is incremented by 2 bytes. Similarly, the value is increased in proportion to their size.
If we used a four-bit machine, the same output would have been incremented by four bytes for integer data.
Note: also read about the Pointer to Pointer in C
Follow Me
If you like my post please follow me to read my latest post on programming and technology.
https://www.instagram.com/coderz.py/
https://www.facebook.com/coderz.py
Staying up to the mark is what defines me. Hi all! I’m Rabecca Fatima a keen learner, great enthusiast, ready to take new challenges as stepping stones towards flying colors.
Leave a Comment
You must be logged in to post a comment.