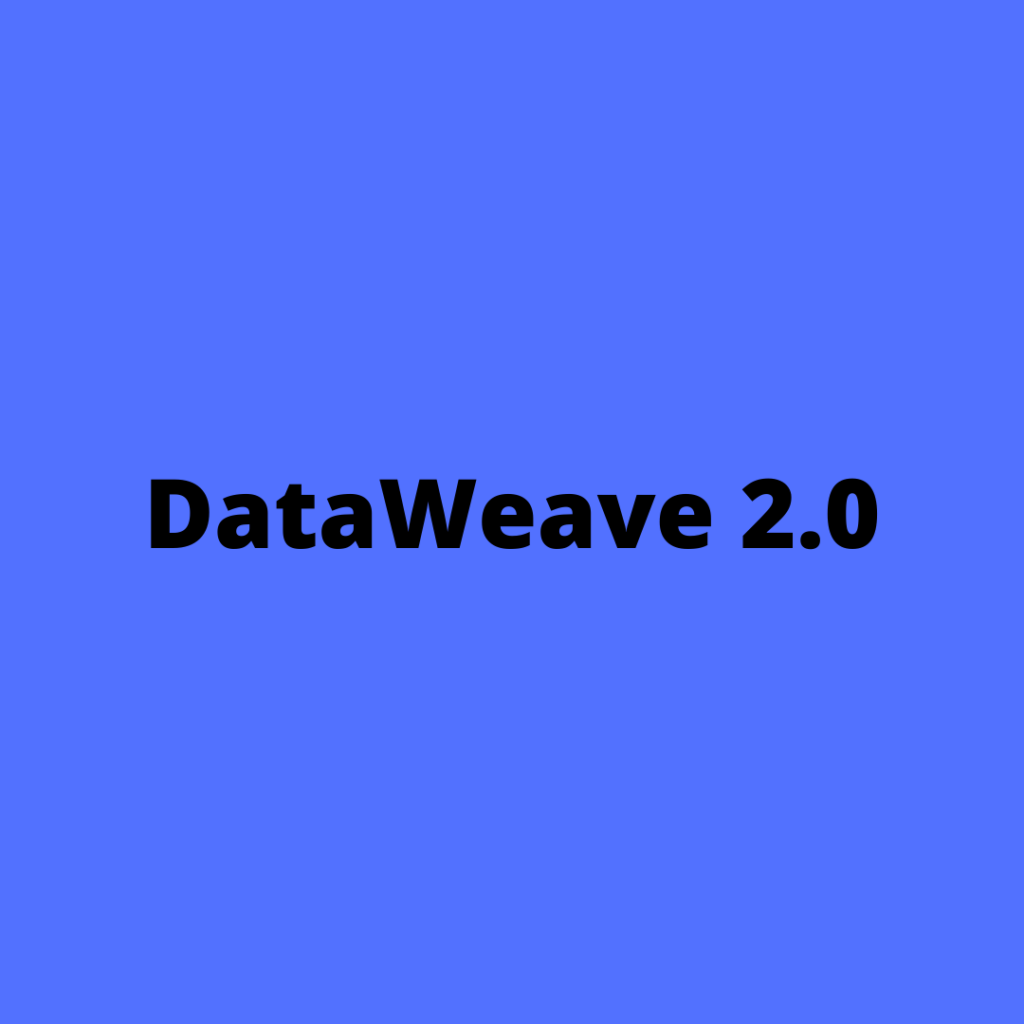
Use selector expressions with the update
operator to specify the fields to update.
Value Selector
This matching selector can check for matches to any value within an object.
The following example updates a field at the root level and a field in a nested level using the value selector:Source
%dw 2.0
var myInput = {
"name": "Ken",
"lastName":"Shokida",
"age": 30,
"address": {
"street": "Amenabar",
"zipCode": "AB1234"
}
}
output application/json
---
myInput update {
case age at .age -> age + 1
case s at .address.street -> "First Street"
}
Output
{
"name": "Ken",
"lastName": "Shokida",
"age": 31,
"address": {
"street": "First Street",
"zipCode": "AB1234"
}
}
Index Selector
This matching selector enables you to match any array by its index.
The following example updates an element that is at the first location of the array:Input
{
"name": "Ken",
"lastName":"Shokida",
"age": 30,
"addresses": [{
"street": "Amenabar",
"zipCode": "AB1234"
}]
}
Source
%dw 2.0
output application/json
---
payload update {
case s at .addresses[0] -> {
"street": "Second Street",
"zipCode": "ZZ123"
}
}
Output
{
"name": "Ken",
"lastName": "Shokida",
"age": 30,
"addresses": [
{
"street": "Second Street",
"zipCode": "ZZ123"
}
]
}
Attribute Selector
This matching selector enables you to match any attribute value.
The following example updates an attribute value and changes it to upper case:Input
<user name="leandro"/>
Source
%dw 2.0
output application/json
---
payload update {
case name at .user.@name -> upper(name)
}
Output
<?xml version='1.0' encoding='UTF-8'?>
<user name="LEANDRO"/>
Dynamic Selector
Any selector can incorporate an expression to make the selection dynamic.
The following example dynamically selects and updates the value of a field that is not hardcoded. Such a field can change at runtime. The example uses the variable theFieldName
to obtain the field with key name
, and it changes the value of that field to Shoki
.Input
{
"name": "Ken",
"lastName":"Shokida"
}
Source
%dw 2.0
var theFieldName = "name"
output application/json
---
payload update {
case s at ."$(theFieldName)" -> "Shoki"
}
Output
{
"name": "Shoki",
"lastName": "Shokida"
}
Conditional Update
The update
operator has syntax for writing a conditional update if you want to modify a field under a given condition.
The following example updates the category based on the name of the user:Input
[{"name": "Ken", "age": 30}, {"name": "Tomo", "age": 70}, {"name": "Kajika", "age": 10}]
Source
%dw 2.0
output application/json
---
payload map ((user) ->
user update {
case name at .name if(name == "Ken") -> name ++ " (Leandro)"
case name at .name if(name == "Tomo") -> name ++ " (Christian)"
}
)
Output
[
{
"name": "Ken (Leandro)",
"age": 30
},
{
"name": "Tomo (Christian)",
"age": 70
},
{
"name": "Kajika",
"age": 10
}
]
Upserting
The update
operator enables you to insert a field that is not present already by using the !
symbol at the end of the selector expression. When the field is not present, the operator binds a null
value to the variable.
The following example updates each object in the array myInput
. If the field name
is present in the object, the example applies the upper
function to the value of name
. If the field name
is not present in the object, the example appends the key-value pair "name": "JOHN"
to the object.Source
%dw 2.0
var myInput = [{"lastName": "Doe"}, {"lastName": "Parker", "name": "Peter" }]
output application/json
---
myInput map ((value) ->
value update {
case name at .name! -> if(name == null) "JOHN" else upper(name)
}
)
Output
[
{
"lastName": "Doe",
"name": "JOHN"
},
{
"lastName": "Parker",
"name": "PETER"
}
]
Sugar Syntax
The update
operator accepts a default variable named $
in place of the longer <variable_name> at
syntax.
The following example binds the value of the expression .age
to the default variable name $
, which is used to express the new value. The longer equivalent is shown in comments.Input
{
"name": "Ken",
"lastName":"Shokida",
"age": 30,
"address": {
"street": "Amenabar",
"zipCode": "AB1234"
}
}
Source
%dw 2.0
output application/json
---
payload update {
//equivalent expression:
//case age at .age -> age + 1
case .age -> $ + 1
case .address.street -> "First Street"
}
Output
{
"name": "Ken",
"lastName": "Shokida",
"age": 31,
"address": {
"street": "First Street",
"zipCode": "AB1234"
}
}
Follow Me
If you like my post please follow me to read my latest post on programming and technology.

Leave a Comment
You must be logged in to post a comment.