
In Java, a thread can be in any of the following states at any given time. A thread is only ever in one of the states depicted:
- New
- Runnable
- Blocked
- Waiting
- Timed Waiting
- Terminated
Life cycle of a Thread diagram:
The diagram shown below represents various states of a thread at any instant in time.
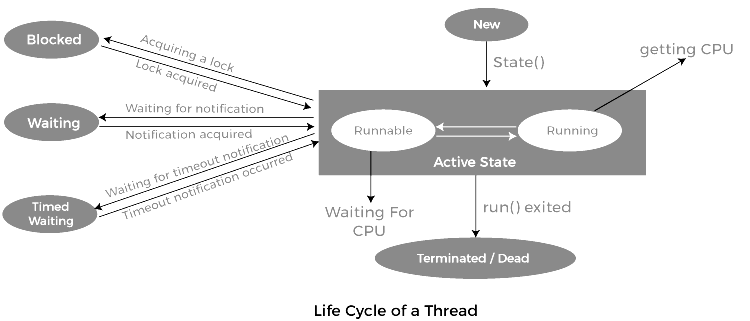
The life cycle of a Thread:
- New Thread: A new thread is in its new state when it is first created. When the thread is in this state, it has not yet begun to run. A thread’s code has not yet run and has not begun to execute when it is in the new state.
- Runnable State: When a thread is prepared to execute, it is transferred to this state. A thread may be actively running in this state, or it may be prepared to begin at any moment. The thread scheduler is in charge of giving the thread enough time to run.
Each thread in a multithreaded program receives a fixed amount of time. Every thread runs for a brief period of time before pausing and handing over the CPU to another thread so that others can have a chance to run. When this occurs, the thread that is currently running and all such threads that are waiting for the CPU is in a runnable state. - Blocked/Waiting state: A thread is in one of the following states when it is momentarily inactive: Blocked/Waiting
- Timed Waiting: When a thread calls a method with a time-out parameter, it enters a timed waiting state. Until the timeout expires, or a notification is received, a thread is in this state. For instance, a thread enters a timed waiting state when it calls sleep or a conditional wait.
- Terminated State: A thread can end for any of the following reasons:
Because it exits normally. This happens when the code of the thread has been entirely executed by the program. Because there occurred some unusual erroneous event, like segmentation fault or an unhandled exception.
Implementation of Thread States
The Thread.getState() method in Java can be used to retrieve the thread’s current state. Java.lang is offered by Java. Below is a summary of the Thread.State class, which defines the ENUM constants for a thread’s state:
1. New
Declaration: public static final Thread.State NEW
Description: Thread state for a thread that has not yet started.
2. Runnable
Declaration: public static final Thread.State RUNNABLE
Description: Thread state for a runnable thread. The Java virtual machine is currently running a thread in the runnable state, but it might be awaiting additional resources from the operating system, like a processor.
3. Blocked
Declaration: public static final Thread.State BLOCKED
Description: Thread state for a thread blocked waiting for a monitor lock. In order to enter a synchronized block or method or to reenter a synchronized block or method after calling object, a thread in the blocked state must first obtain a monitor lock. wait().
4. Waiting
Declaration: public static final Thread.State WAITING
Description: Thread state for a waiting thread. Thread state for a waiting thread. A thread is in the waiting state due to calling one of the following methods:
- Object.wait with no timeout
- Thread.join with no timeout
- LockSupport.park
5. Timed Waiting
Declaration: public static final Thread.State TIMED_WAITING
Description: Thread state for a waiting thread with a specified waiting time.When one of the methods listed below is called with a positive waiting time specification, a thread enters the timed waiting state:
- Thread.sleep
- Object.wait with timeout
- Thread.join with timeout
- LockSupport.parkNanos
- LockSupport.parkUntil
6. Terminated
Declaration: public static final Thread.State TERMINATED
Description: Thread state for a terminated thread. The thread has completed execution.
Example:
// Java program to demonstrate thread states
class thread implements Runnable {
public void run()
{
// moving thread2 to timed waiting state
try {
Thread.sleep(1500);
}
catch (InterruptedException e) {
e.printStackTrace();
}
System.out.println(
"State of thread1 while it called join() method on thread2 -"
+ Test.thread1.getState());
try {
Thread.sleep(200);
}
catch (InterruptedException e) {
e.printStackTrace();
}
}
}
public class Test implements Runnable {
public static Thread thread1;
public static Test obj;
public static void main(String[] args)
{
obj = new Test();
thread1 = new Thread(obj);
// thread1 created and is currently in the NEW
// state.
System.out.println(
"State of thread1 after creating it - "
+ thread1.getState());
thread1.start();
// thread1 moved to Runnable state
System.out.println(
"State of thread1 after calling .start() method on it - "
+ thread1.getState());
}
public void run()
{
thread myThread = new thread();
Thread thread2 = new Thread(myThread);
// thread1 created and is currently in the NEW
// state.
System.out.println(
"State of thread2 after creating it - "
+ thread2.getState());
thread2.start();
// thread2 moved to Runnable state
System.out.println(
"State of thread2 after calling .start() method on it - "
+ thread2.getState());
// moving thread1 to timed waiting state
try {
// moving thread1 to timed waiting state
Thread.sleep(200);
}
catch (InterruptedException e) {
e.printStackTrace();
}
System.out.println(
"State of thread2 after calling .sleep() method on it - "
+ thread2.getState());
try {
// waiting for thread2 to die
thread2.join();
}
catch (InterruptedException e) {
e.printStackTrace();
}
System.out.println(
"State of thread2 when it has finished it's execution - "
+ thread2.getState());
}
}
Output:
State of thread1 after creating it - NEW
State of thread1 after calling .start() method on it - RUNNABLE
State of thread2 after creating it - NEW
State of thread2 after calling .start() method on it - RUNNABLE
State of thread2 after calling .sleep() method on it - TIMED_WAITING
State of thread1 while it called join() method on thread2 -WAITING
State of thread2 when it has finished it's execution - TERMINATED
Explanation: When a new thread is created, the thread is in the NEW state. A thread is moved to the Runnable state by the thread scheduler when its start() method is called. The thread currently processing that statement will wait for that thread to enter the Terminated state whenever the join() method is called on a thread instance. The program then calls join() on thread2, making thread1 wait while thread2 completes its execution and transitions to the Terminated state before the final statement is printed on the console. Because it called join() on thread2, thread1 enters the waiting state as it waits for thread2 to finish running.
Note: also read about the Multithreading in Java
Follow Me
If you like my post, please follow me to read my latest post on programming and technology.
https://www.instagram.com/coderz.py/
https://www.facebook.com/coderz.py
Staying up to the mark is what defines me. Hi all! I’m Rabecca Fatima a keen learner, great enthusiast, ready to take new challenges as stepping stones towards flying colors.
Leave a Comment
You must be logged in to post a comment.