
JDBC (Java Database Connectivity) is the Java API for connecting to a database, issuing queries and commands, and handling database result sets. It is a component of JavaSE (Java Standard Edition). JDBC API connects to the database using JDBC drivers. JDBC is fundamentally a specification that provides a comprehensive set of interfaces for mobile access to an underlying database.
Applications of JDBC:
Java can be used to create a variety of executables, including
- Java Applications
- Java Applets
- Java Servlets
- Java Server Pages (JSP)
- Enterprise JavaBeans (EJB).
JDBC 4.0 Packages:
The two main packages for JDBC 4.0 are java.sql and javax.sql. The following changes are among the new features in these packages:
- automatic loading of the database driver
- Exception handling enhancements.
- BLOB/CLOB functionality improvements.
- Improvements to the connection and statement interface.
- support for a country’s character set.
- ROWID access in SQL.
- Support for XML data types in SQL 2003
- Annotations.
JDBC 4.1 packages:
The following features are introduced by JDBC 4.1, which is a component of Java SE 7:
- the capacity to utilize a try-with-resources statement to force Connection, ResultSet, and Statement resources to close automatically
- RowSet 1.1: The creation of all sorts of row sets that are supported by your JDBC driver thanks to the inclusion of the RowSetFactory interface and the RowSetProvider class.
JDBC 4.2 packages:
The following features are included in JDBC 4.2, which is a component of Java SE 8:
- Support for REF CURSOR has been added.
- Java.sql has been added.
- Interface for DriverAction
- adding the java.sql file.
- Interface for SQL
- JDBCType Enum has been added in Java.
- Add assistance for high update rates
- alterations to the current interfaces
- Rowset 1.2: A list of JDBC RowSet’s improvements.
Architecture of JDBC:
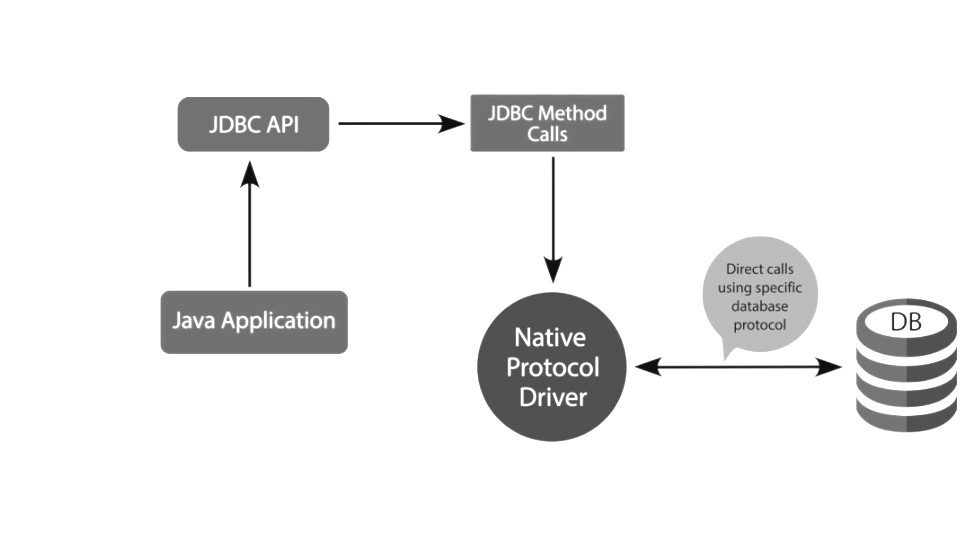
- Application: It connects to a data source via a java applet or servlet.
- The JDBC API: Java programs can run SQL commands and get results using the JDBC API.
- DriverManager:In the JDBC architecture, it is significant. Enterprise applications are successfully connected to databases using some drivers designed specifically for databases.
- JDBC drivers: A JDBC driver that interacts intelligently with the relevant data source is required in order to communicate with a data source using JDBC.
Drivers for JDBC:
JDBC drivers are client-side adapters that translate requests from Java programs into a protocol that the DBMS can comprehend. They are installed on the client machine rather than the server. JDBC drivers come in four varieties:
- JDBC-ODBC bridge driver, type-1 driver
- Native-API driver or Type-2 driver
- Network protocol driver, or Type-3 driver
- Thin driver or Type-4 driver
Bridge JDBC-ODBC driver:
The JDBC-ODBC bridge driver connects to the database using an ODBC driver. JDBC method calls are transformed into ODBC function calls by the JDBC-ODBC bridge driver. Due to thin drivers, this practice is currently discouraged.
Advantages | Disadvantages |
simple to use. | Performance degraded because JDBC method call is converted into the ODBC function calls. |
connects to any database with ease. | The client computer has to have the ODBC driver installed. |
Native-API driver:
The client-side libraries of the database are used by the Native API driver. The driver transforms calls to JDBC methods into native API calls for the database. It is not completely written in Java.
Advantages | Disadvantages |
Better performance than JDBC-ODBC bridge driver. | The Native driver must be installed on each client PC, which is a drawback. |
On the client computer, the vendor client library must be installed. |
Network protocol drivers:
Indirectly or directly, the Network Protocol Driver uses middleware (an application server) to translate JDBC calls into the vendor-specific database protocol. It is entirely written in Java.
Advantages | Disadvantages |
Because the application server can handle numerous duties like auditing, load balancing, logging, etc., there is no need for a client side library. | Client machines must have network support. |
requires middle-tier code for databases specifically. | |
Due to the middle tier’s need for database-specific coding, Network Protocol driver maintenance becomes expensive. |
Thin driver:
Direct conversion of JDBC calls into vendor-specific database protocol is performed by the thin driver. It is called a “thin driver” for this reason. It is entirely written in Java.
Advantages | Disadvantages |
greater efficiency compared to all other drives. | Neither the client side nor the server side require any software. |
Drivers rely on the Database, which is a disadvantage. |
DriverManager Class:
The basic service to set of JDBC drivers is managed by the DriverManager class. It serves as an interface between drivers and Java applications. The “jdbc.drivers” system attribute references several driver classes, which the DriverManager class will attempt to load.
Methods of DriverManager Class:
Methods | Description |
---|---|
getDriver(String url) | Helps to locate a driver that understands the given URL. |
registerDriver(Driver driver) | Used to register the given driver with the DriverManager class. |
static void deregisterDriver(Driver driver) | Removes the specified driver from the DriverManager class. |
static Connection getConnection(String url) | It creates the connection with the given database URL. |
static Connection getConnection(String url, String username, String password) | Establishes the connection with the given database URL with username and password. |
Connection method:
A Java application and a database are in a session known as a connection. The connection to the database is made easier with it.
Method | Description |
---|---|
public Statement createStatement() | For the purpose of running SQL queries, it is used to create an object of the statement. |
public Statement createStatement(intresultSetType,intresultSetConcurrency) | It is utilized to generate objects for the ResultSet based on the specified type and concurrency. |
public void setAutoCommit(boolean status) | For setting the commit status, use this. By default, it is always true. |
public void commit() | It is used to save the changes which have been commit or rollback permanent |
public void rollback() | It is used to delete the changes which have been commit or rollback permanent |
public void close() | It is used to delete the changes which have been commit or rollback permanent |
Statement interface:
The Statement interface offers ways to use the database to run queries. The statement interface functions as a factory for ResultSets, offering factory methods to obtain ResultSet objects.
Method | Description |
public ResultSet executeQuery(String sql) | is used to execute SELECT query and return the object of ResultSet. |
public int executeUpdate(String sql) | is used to execute specified query, it may be create, drop, insert, update, delete, etc. |
public boolean execute(String sql) | is used to execute queries that may return multiple results. |
public int[] executeBatch() | is used to execute a batch of commands. |
ResultSet interface:
The ResultSet object keeps a cursor pointed at a table row. The cursor initially points before the first row.
public boolean next(): | moves the cursor to the row that is one above the current row. |
public boolean previous(): | moves the cursor to the one row previous from the current position. |
public boolean first(): | moves the cursor to the first row in result set object. |
public boolean last(): | moves the cursor to the last row in result set object. |
public boolean absolute(int row): | moves the cursor to the specified row number in the ResultSet object. |
public boolean relative(int row): | moves the cursor to the relative row number in the ResultSet object, it may be positive or negative. |
public int getInt(int columnIndex): | returns the data of the specified column index of the current row as int. |
public int getInt(String columnName): | returns the data of the specified column name of the current row as int. |
public String getString(int columnIndex): | returns the data of the specified column index of the current row as String. |
public String getString(String columnName): | return the data of the specified column name of the current row as String. |
PreparedStatements interface:
The Statement interface has a subinterface called PreparedStatement. It’s employed to run parameterized queries.
Method | Description |
---|---|
public void setInt(int paramIndex, int value) | the integer value is changed to the specified parameter index. |
public void setString(int paramIndex, String value) | the String value is changed to the specified parameter index. |
public void setFloat(int paramIndex, float value) | the float value is changed to the specified parameter index. |
public void setDouble(int paramIndex, double value) | the double value is changed to the specified parameter index. |
public int executeUpdate() | executes the query. It is used for create, drop, insert, update, delete etc. |
public ResultSet executeQuery() | executes the select query. It returns an instance of ResultSet. |
Note: also read about the Remote Method Invocation(RMI) in Java
Follow Me
If you like my post, please follow me to read my latest post on programming and technology.
https://www.instagram.com/coderz.py/
https://www.facebook.com/coderz.py
Staying up to the mark is what defines me. Hi all! I’m Rabecca Fatima a keen learner, great enthusiast, ready to take new challenges as stepping stones towards flying colors.
Leave a Comment
You must be logged in to post a comment.