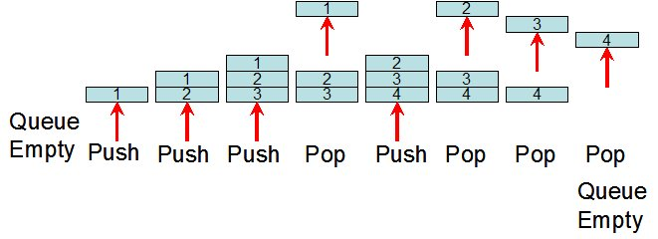
Overview of Queue Data Structure 🤔🤓
- A queue is an ordered collection of items where the addition of new items happens at one end, called the “rear,” and the removal of existing items occurs at the other end, commonly called the “front.”
- As an element enters the queue it starts at the rear and makes its way toward the front, waiting until that time when it is the next element to be removed.
- The most recently added item in the queue must wait at the end of the collection. ¨The item that has been in the collection the longest is at the front.
- This ordering principle is sometimes called FIFO, first-in-first-out. 👈😲
- It is also known as “first-come-first-served.” 👈😍
- The simplest example of a queue is the typical line that we all participate in from time to time.
- We wait in a line for a movie, we wait in the check-out line at a grocery store, and we wait in the cafeteria line.
- The first person in that line is also the first person to get serviced/helped.
- Note how we have two terms here, Enqueue and Dequeue. 🤗✌️
- The enqueue term describes when we add a new item to the rear of the queue.
- The dequeue term describes removing the front item from the queue.
- You should now have a basic understanding of Queues and the FIFO principal for them. 👌👌
Now we are going to implement our own Queue class 👇👇
class Queue(object): def __init__(self): self.items = [] def isEmpty(self): return self.items == [] def enqueue(self, data): return self.items.insert(0, data) def dequeue(self): return self.items.pop() def size(self): return len(self.items) q = Queue() print("Is Empty {}".format(q.isEmpty())) q.enqueue(1) q.enqueue(2) q.enqueue(3) print(q.dequeue()) print("Size {}".format(q.size()))
Recommended Reading:
How to start learning Python Programming 👈
Implementation of Stack in Python 👈
Follow Me ❤😊
If you like my post please follow me to read my latest post on programming and technology.
Leave a Comment
You must be logged in to post a comment.