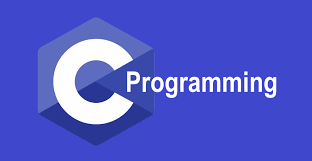
A pointer variable stores the address of another pointer variable; this is referred to as a pointer to a pointer variable in C.
It is a type of multiple indirections, also known as a chain of pointers. A pointer contains the address of a variable, and when a pointer to a pointer is defined, the first pointer contains the address of the second pointer, which points to the actual variable’s address.
Let us understand this better with the help of an example:
int b=10;
int *p=&b;
int **ptr=&p;
here:
- a variable b has a value of 10,
- p is an integer pointer that points to b i.e, stores the address of variable b
- then we have another integer pointer ptr which points towards pointer p, i.e, stores the memory location of pointer p.
Using ptr we can now access the pointer p as well as the value of variable b.
Example:
#include<stdio.h>
int main()
{
int b;
int *p;
int **ptr;
b = 10;
/* take the address of b */
p = &b;
/* take the address of p using address of operator & */
ptr = &p;
/* take the value using ptr */
printf("Value of b = %d\n", b );
printf("Value available at *p = %d\n", *p );
printf("Value available at **ptr = %d\n", **ptr);
/* take the address */
printf("Value of &b = %u\n", &b );
printf("Value available at p = %u\n", p );
printf("Value available at &p = %u\n", &p );
printf("Value available at &ptr = %u\n", &ptr);
printf("Value available at ptr = %u\n", ptr);
printf("Value available at *ptr = %u\n", *ptr);
return 0;
}
Output:
Value of b = 10
Value available at *p = 10
Value available at **ptr = 10
Value of &b = 2051687924
Value available at p = 2051687924
Value available at &p = 2051687928
Value available at &ptr = 2051687936
Value available at ptr = 2051687928
Value available at *ptr = 2051687924
Note: also read about the Pointer to structure
Follow Me
If you like my post please follow me to read my latest post on programming and technology.
https://www.instagram.com/coderz.py/
https://www.facebook.com/coderz.py
Staying up to the mark is what defines me. Hi all! I’m Rabecca Fatima a keen learner, great enthusiast, ready to take new challenges as stepping stones towards flying colors.
Leave a Comment
You must be logged in to post a comment.