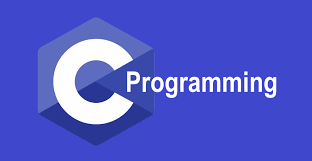
The response and recovery procedures from error conditions present in a software application are referred to as error handling.
C programming does not provide direct error handling support, but as a system programming language, it does provide access at a lower level in the form of return values.
In the event of an error, most C or Unix function calls return -1 or NULL and set the error code errno. It is declared as a global variable and indicates that an error occurred during any function call. The error.h> header file defines various error codes.
strerror, errno, and perror() ()
The C programming language includes perror() and strerror() functions for displaying the text message associated with errno.
- The perror() function returns the string you passed it, followed by a colon, a space, and the textual representation of the current errno value.
- The strerror() function, returns a pointer to the current errno value’s textual representation.
Example:
#include <stdio.h>
#include <errno.h>
#include <string.h>
extern int errno ;
int main () {
FILE * pf;
int errnum;
pf = fopen ("file.txt", "rb");
if (pf == NULL) {
errnum = errno;
fprintf(stderr, "Value of errno: %d\n", errno);
perror("Error printed by perror");
fprintf(stderr, "Error opening file: %s\n", strerror( errnum ));
} else {
fclose (pf);
}
return 0;
}
Output:
Value of errno: 2
Error printed by perror: No such file or directory
Error opening file: No such file or directory
Divide by Zero Errors
It is a common problem that when dividing any number, programmers do not check to see if the divisor is zero, resulting in a runtime error.
The code below corrects this by first checking to see if the divisor is zero before dividing.
#include <stdio.h>
#include <stdlib.h>
main() {
int dividend = 20;
int divisor = 0;
int quotient;
if( divisor == 0){
fprintf(stderr, "Division by zero! Exiting...\n");
exit(-1);
}
quotient = dividend / divisor;
fprintf(stderr, "Value of quotient : %d\n", quotient );
exit(0);
}
Output:
Division by zero! Exiting...
Exit Status:
The C standard defines two constants, EXIT SUCCESS and EXIT FAILURE, which can be passed to exit() to indicate a successful or unsuccessful termination. These are macros defined in the header file stdlib.h.
#include <stdio.h>
#include <errno.h>
#include <string.h>
#include <stdlib.h>
int main ()
{
FILE * fp;
fp = fopen ("filedoesnotexist.txt", "rb");
if (fp == NULL)
{
printf("Value of errno: %d\n", errno);
printf("Error opening the file: %s\n",
strerror(errno));
perror("Error printed by perror");
exit(EXIT_FAILURE);
printf("I will not be printed\n");
}
else
{
fclose (fp);
exit(EXIT_SUCCESS);
printf("I will not be printed\n");
}
return 0;
}
Output:
Value of errno: 2
Error opening the file: No such file or directory
Error printed by perror: No such file or directory
Note: also read about the File handling in C
Follow Me
If you like my post please follow me to read my latest post on programming and technology.
https://www.instagram.com/coderz.py/
https://www.facebook.com/coderz.py
Staying up to the mark is what defines me. Hi all! I’m Rabecca Fatima a keen learner, great enthusiast, ready to take new challenges as stepping stones towards flying colors.
Leave a Comment
You must be logged in to post a comment.